Python
Introduction
This document describes the usage of the "Script"-Filter which was introduced with the dab:Exporter version 3.0.112. With this filter you can provide Python scripts with which filter values can be derived. The purpose of this document is to give an overview of the possibilities as well as showing some filter examples.
Mode of Operation
In the package management a filter with the filter clause "Script" can be defined. This filter clause only works with the field origins "input" and "scheduler". At the filter field definitions all data types can be chosen but you must only add one filter field definition per script filter.
The Python script will be invoked with three variables during the download. These variables are
- the field mapping
- "from date" (input / computation of scheduler)
- "to date" (input / computation of scheduler)
The Python script has to store the return value in a variable as a string which has to be a valid ABAP statement and has to match the following pattern "FIELDNAME = ´VALUE´".
Example:
"MANDT = ´800´"
The provided script will be evaluated for every entered date range (multiple ranges are possible) / scheduler computation per field mapping per table separately.
Warning: If the script returns a constant value like in the example above and the script will be evaluated more than once because of manually entered multiple date ranges then the records will also be extracted multipletimes.
Package management filter settings
The Python script filter can be created just like you are used to.
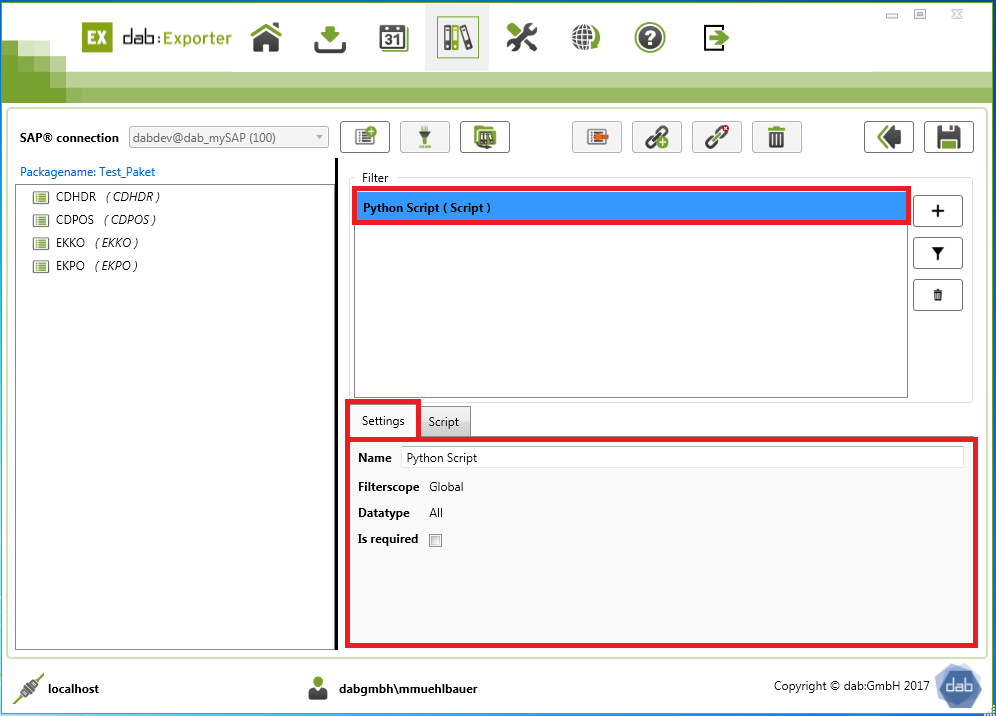
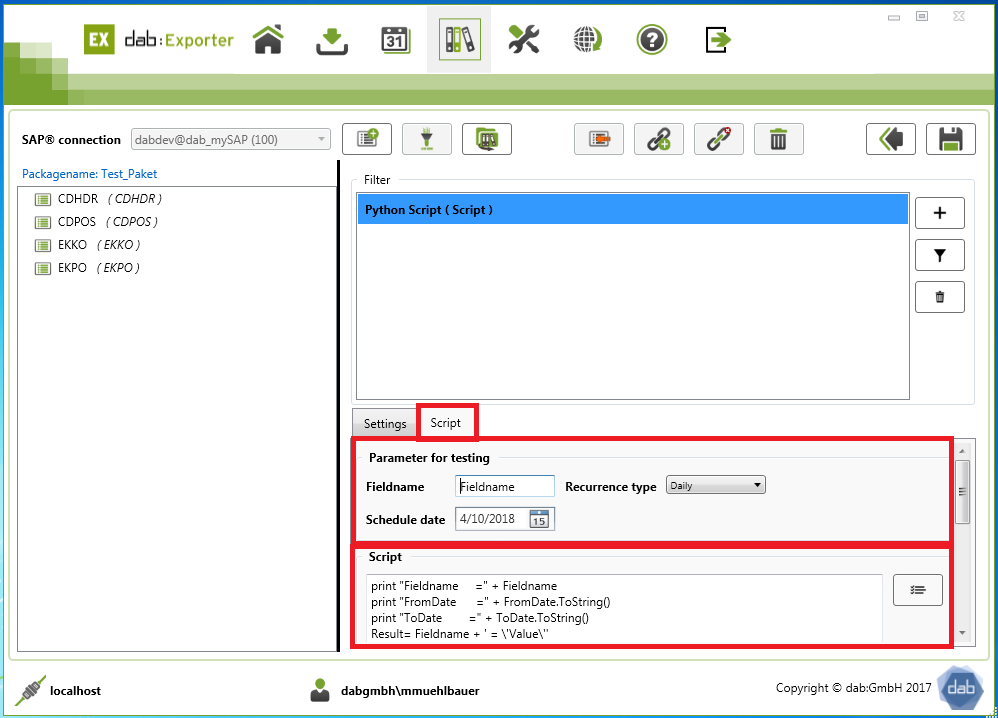
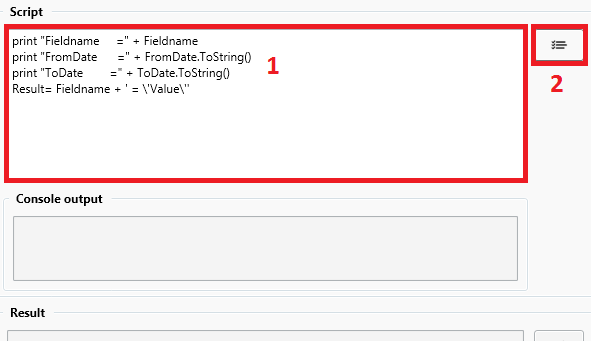
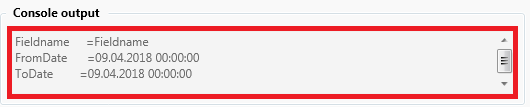

In the area "Parameter for testing" you can adjust for developing the script, the values of the variables with which the script will be invoked.
The fieldname corresponds to the name of the field on which the filter is mapped. The recurrence type changes the computation of the date ranges based on the schedule date.
The script area is divided in two areas. In top area the Python script has to be entered. If you open the script window of the filter for the first time it will be filled with a default script. This default script prints all given variables to the console and returns the "Result" variable accordingly. You have to place your valid ABAP code within this variable. The lower area server for displaying the output of the console of the Python interpreter (print statements) after clicking on the button "Eval". The result of the script evaluation or errors will be displayed in the "Result" area. After a successful script evaluation you can apply the script to the filter which means you can only "Apply" a script to a filter if it was evluated succesfully and only if you wish to apply it. With the button "Close" you will retrun to the filter overview.
1Even if times are displayed you can only use date values for the computation! You must not rely on the correctness of the time values!
2This value will not be provided by the scheduler. It only server for simulating user input
3Only values for Daily "every day" will be computed
Advices for creating scripts
With the "Script" filter you are able to create complex filters with the Python programming language. The implementation does not restrict you in any way which means you have access to a full programming language.
The implementation of the script evaluation expects the script to terminate in a finite time which means infinite loops or script errors can crash the program (or consume a download slot permanently - until a restart of the server). Moreover it is not recommended to access any kind of foreign resources which include but are not limited to files or network resources. Furthermore you should not start any processes or threads! Also you must not open any "Windows" or require any user input.
Python Implementation
In the dab:Exporter the .NET Implementation (IronPython 2.7.4 ) of Python is used. The Python version is 2.6. Basically any additional documentation for Python 2.6 can be used for this IronPython version:
- http://www.tutorialspoint.com/python/
- https://docs.python.org/2.6/
- http://ironpython.net/documentation/
Because this python version is written in .NET you can access the whole .NET framework if you need to. The variables “FromDate” as well as “ToDate” are .NET structures (System.DateTime).
Short Python Tutorial
Python is an interpreted dynamically typed higher level programming language. In this programming language each instruction has to be written in an extra line. Moreover the code must not be structured like in C-like languages with curly braces but with indentation exclusively. The indentation can be done with spaces or tabs but the indentation has to be consistent within a script. In the following examples tabs are used for indentation and is recommended for creating scripts.
Console Outputs
With the print
statement you can write values to the console. Following examples prints "Hello World!"
two times on the console.
print "Hello World!"
a = "Hello World!"
print a
During the development of a script filter you can add print statements any time to display the value of a variable. The output of the console are not evaluated by the dab:Exporter and should only be used during the script development.
Comments
Comments start with a „#“ and are valid until the end of the line. Following example prints “Hello World!” two times:
# comment print "Hello World!"
a = "Hello World!" # additional comment
print a
Variable names / identifier
Variables / Identifier are case-sensitive and have to start with a letter (A-Za-z) or an underscore (_). Then you can use letters, numbers or more underscores. You may not use the chars @,$ and %.
_ = "Hello World!"
a = "Hello a"
A = "Hello big a"
print _
print a
print A
Console:
Hello World!
Hello a
Hell big a
The following table shows reserved words of the Python programming language and can not be used as variable names / identifier:
Table of reserverd words of Python | ||||
and | assert | brea | class | continue |
def | del | elif | else | except |
exec | finally | for | from | global |
if | import | in | is | lambda |
not | or | pass | raise | |
return | try | while | with | yield |
Datatypes
Strings are enclosed in single or double quotes in Python you just have to use the same char for ending the string. If you want to use the char within a string with which you want to enclose the string you have to escape it with a backslash.
a = "hello"
b = 'hell'
c = "\""
d = '\''
Console:
hello
hello
"
'
Strings can be concatenated with +:
a = "hello"
b = 'world'
print a + " " + b
Console:
hello world
Numbers can be casted to strings with the function “str”. Strings can be converted to an integer number with the function “int“ or with the function “float“ to a floating point number. A type conversion from an integer to a floating point number is automatically done by python..
a = int("5")
b = float(str(2.5))
c = a + b
d = a / b
e = (float("2.5") + 2.5) * 2 / int(str(4))
print c
print d
print e
Console:
7.5
2.0
2.5
Control Structures
A conditional statement is written in Python like this
year = 2014
if (year == 2014):
print "year is 2014"
Console:
year is 2014
If you have more than one condition you want to check on the structure looks like this (tab is used as indentation)
year = 2013
if year == 2012:
print "year is 2012"
elif year == 2013:
print "year is 2013"
elif year == 2014:
print "year is 2014"
else:
print "year is something else"
Console:
year is 2013
In the example above the "elif" conditions are optional. Moreover within each condition you can have any other code structure.
Functions
Functions have to be defined before they are called. The result of a function is returned with the “return“ statement which also terminates the function. Calling the “return” statement without a value returns nothing.
def add(param1, param2):
return param1 + param2
print add("Hello", "World")
print add(1,2)
Console:
Hello World
3
More String Functions
It is possible to create formatted strings with placeholders which can be evaluated with variables. For this you have to create a string containing a %-symbol and a formatting statement. After the string you have to use another %-symbol and the variables you want to use.
print "%s = '%s'" % ('MANDT', 800)
Console:
MANDT = '800'
Following formatting statements can be used:
Formatting Symbol | Conversion |
%c | Character |
%s | str function call |
%i | signed integer |
%d | signed integer |
%u | unsigned integer |
%o | octal integer |
%x | hexadecimal integer (lowercase) |
%X | hexadecimal integer (uppercase) |
%f | floating point number |
You can find more string functions at Tutorialspoint
ABAP - Statement
You have to assign a valid ABAP statement to the variable “Result”. The dab:Exporter handles the correct usage and linking of the filters which means your ABAP statement must not start with an “AND“, “OR“ or any other link operator. But the linking operators can be used within the filter. Following link operators / symbols are recognized by the dab:Exporter:
Filter operations | ||||||
( | ) | AND | OR | BETWEEN | IN | = |
NOT LIKE | LIKE | > | >= / => | < | <= / =< | <> / >< |
You have to place a space before and after each operator!
Filter examples
The fiscal year is computed with the year of the from date minus 1
gjahr = FromDate.Year - 1
resultStr = "%s = '%s'" % (Fieldname, gjahr)
print resultStr
Result = resultStr
Console:
- Fieldname = Fieldname
- Recurrence = Daily
- Schedule date: 11/14/2014
Fieldname = '2013'
Time interval for TCURF_GDATU
The computation is done by substracting the date in the format YYYYMMDD from 99999999.
def convert(param):
formatted = str(param.Year)
formatted += str(param.Month).zfill(2)
formatted += str(param.Day).zfill(2)
return 99999999 - int(formatted)
formattedFrom = convert(FromDate)
formattedTo = convert(ToDate)
resultStr = "%s >= '%s' AND %s <= '%s'" % (Fieldname, formattedFrom, Fieldname, formattedTo)
print resultStr
Result = resultStr
Console output with following parameters F
- Fieldname = Fieldname
- Recurrence = Daily
- Schedule date: 11/14/2014
Fieldname >= '79858886' AND Fieldname <= '79858886'
Please note there is no new line in the line of the variable "resultStr"!